PiCy - Raspberry Pi Cyborg
Now for the second edition of PiCy.
Now that we have our robot we want to give him a task to do, for our first project we will make him draw a picture:
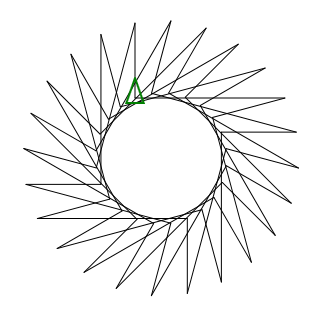
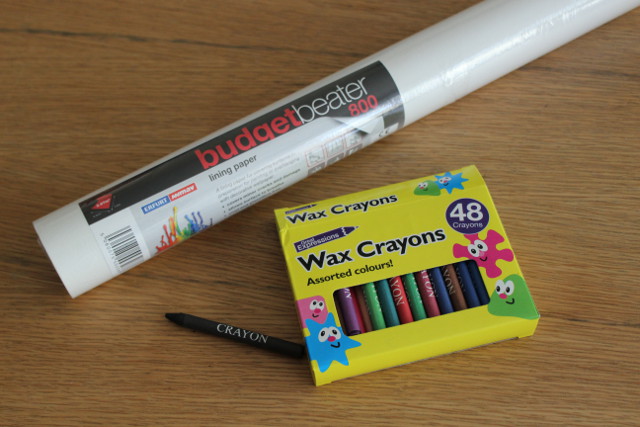
We found that a felt-tipped pen or highlighter work very well for this.
The simplest method is to remove the runner (the leg at the back) and replace it with the pen, which will leave a mark wherever the runner would have been.
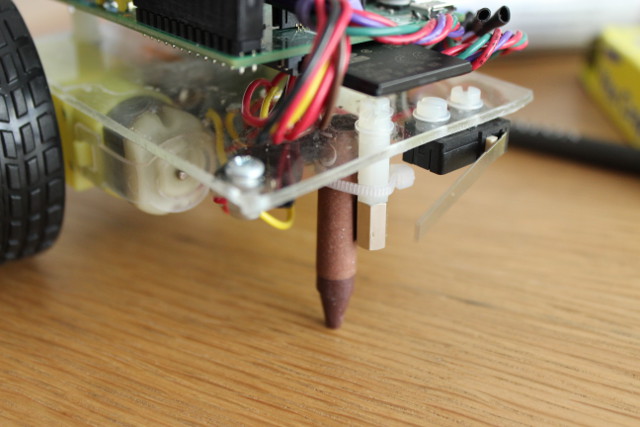
For those who want to draw patterns which involve removing the pen from the page, a solenoid should be connected across drive #3 such that when it is powered the pen touches the paper, and when off the pen is above the paper (you will need to keep the runner and attach the pen elsewhere for this).
What we are going to do is use the TurtleBorg script as a base to build on, giving him a pattern to draw in terms of moving forward and rotating.
Here is the script code:
Make the script executable using
and run using
PiCy should have now just turned in a circle.
If he rotated the wrong amount (not a full circle or too far) you will need to change
Now we have him ready, set him into full running mode by changing line 10 from
Make sure you have plenty of room then run him again with
With any luck PiCy should start drawing a pattern like so:
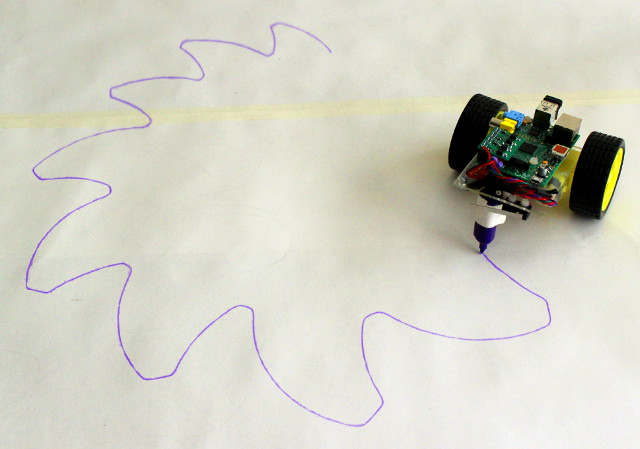
For example to draw a square you could use
and
for the segment.
Since PiCy is being controlled only by time delays you will have to forgive him for a bit of inaccuracy, he will tend to wander over time and we intend to tackle this in a later issue.
Have a go at making your own patterns, but remember bigger patterns need bigger paper :)
Now that we have our robot we want to give him a task to do, for our first project we will make him draw a picture:
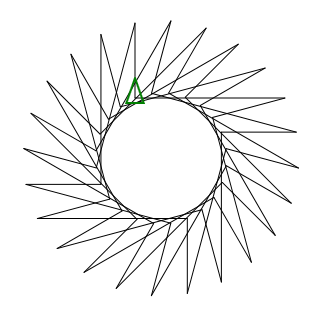
What you will need
- The finished PiCy from last time
- A pen, pencil, or crayon
- Something to attach the above to PiCy
- A very large sheet of paper (plain wallpaper works well)
- A room to run PiCy around in
- The TurtleBorg script, found here
- Optionally a solenoid to control the pen/ pencil / crayon
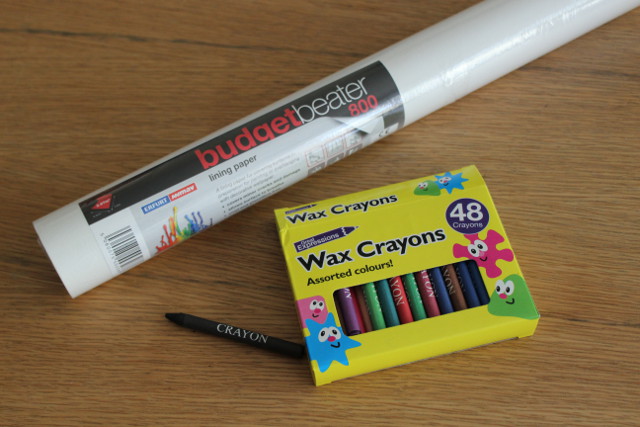
How can we make him draw?
To make PiCy draw we are going to attach a drawing device, such as a pen, to him so wherever he goes he leaves a trail (just like a snail would).We found that a felt-tipped pen or highlighter work very well for this.
The simplest method is to remove the runner (the leg at the back) and replace it with the pen, which will leave a mark wherever the runner would have been.
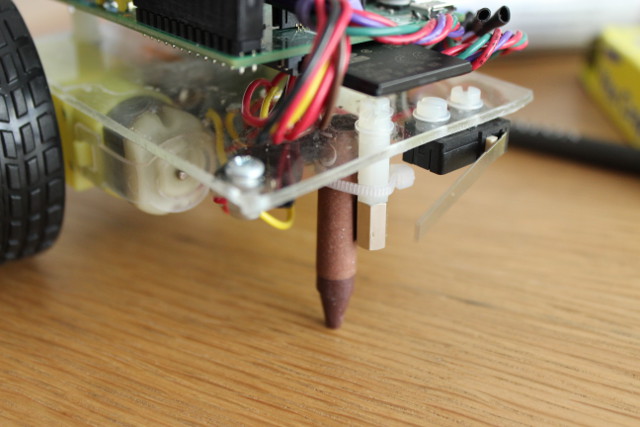
For those who want to draw patterns which involve removing the pen from the page, a solenoid should be connected across drive #3 such that when it is powered the pen touches the paper, and when off the pen is above the paper (you will need to keep the runner and attach the pen elsewhere for this).
How do we tell him what to draw?
This is where the TurtleBorg script (see here) is needed.What we are going to do is use the TurtleBorg script as a base to build on, giving him a pattern to draw in terms of moving forward and rotating.
Here is the script code:
#!/usr/bin/env python # coding: Latin-1 # Load library functions we want import TurtleBorg # Settings for PiCy secondsFullRotate = 2.4 # The number of seconds driving left to rotate full circle (360 deg) runs = 24 # The number of times to repeat the pattern sequence testMode = True # If True run in a mode for tuning secondsFullRotate, if False draw the pattern # Set up PiCy movement functions def Left(angle): TurtleBorg.MoveLeft(angle * (secondsFullRotate / 360.0)) def Right(angle): TurtleBorg.MoveRight(angle * (secondsFullRotate / 360.0)) # Aliases for unchanged functions (local names for the TurtleBorg functions) Forward = TurtleBorg.MoveForward PenDown = TurtleBorg.PenDown PenUp = TurtleBorg.PenUp # Run the sequence if testMode: # Testing mode, perform a 360 degree rotation print 'Testing full circle at %f seconds' % (secondsFullRotate) PenUp(0) Left(360) print 'If PiCy turned too far, reduce secondsFullRotate,' print 'otherwise if PiCy did not turn far enough, increase secondsFullRotate,' print 'finally if PiCy did a full circle then secondsFullRotate is correct' else: # Normal mode print 'Drawing pattern (%d runs)' % (runs) PenDown(0) for i in range(runs): print 'Run %d' % (i + 1) Forward(0.20) Left(165) Forward(0.40) Right(90) print 'All done :)' PenUp(0)Download it here as text and save as PiCyDraw.py on the Raspberry Pi in the same place as TurtleBorg.py.
Make the script executable using
and run using
sudo ./PiCyDraw.py
PiCy should have now just turned in a circle.
If he rotated the wrong amount (not a full circle or too far) you will need to change
secondsFullRotate
on line 8 as per the on screen instructions until he does (it does not have to be precise, but the closer to a full circle the better the pattern will come out).Now we have him ready, set him into full running mode by changing line 10 from
testMode = True
to testMode = False
, causing him to run the sequence from line 34 onwards instead of turning in a circle.Make sure you have plenty of room then run him again with
sudo ./PiCyDraw.py
With any luck PiCy should start drawing a pattern like so:
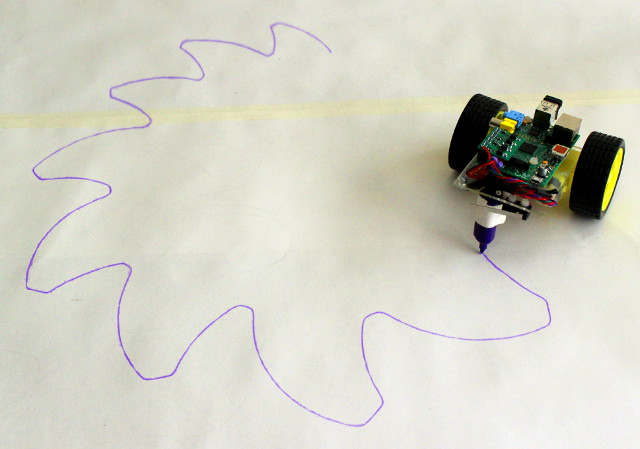
How do I make PiCy draw other things?
Now that you have a drawing you will probably want to make your own drawing, lines 39 to 42 tell him how to draw a single segment of a pattern (a piece to be repeated), and line 9 tells him how many times to run.For example to draw a square you could use
runs = 4
and
Forward(1)
Left(90)
for the segment.
Since PiCy is being controlled only by time delays you will have to forgive him for a bit of inaccuracy, he will tend to wander over time and we intend to tackle this in a later issue.
Have a go at making your own patterns, but remember bigger patterns need bigger paper :)
