DiskMon - Monitor your free space
We recommend using the new driver free based scripts for LedBorg.
The new driver free examples can be found here, the installation can be found here.
We like being able to glance at things and immediately know if they are happy without relying on manually checking.
So when our file server started running out of space we thought it would have been great to be warned.
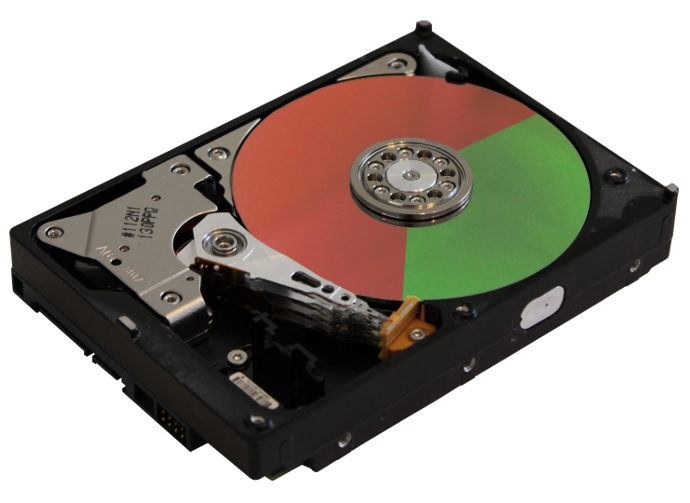
We decided to use an LedBorg to show how full a given mount point (usually a drive or network share),
the colour displayed would be the following:
<=11% | <=22% | <=33% | <=44% | <56% | <67% | <78% | <89% | >=89% |
The result was DiskMon.py, a Python script which reads how full a mount point is and controls an LedBorg appropriately.
Here's the code, you can download the DiskMon script file as text here
Save the text file on your pi as DiskMon.py
Make the script executable using
chmod +x DiskMon.py
and run using
sudo ./DiskMon.py
to monitor the default mount ('/', the SD card itself), or
sudo ./DiskMon.py mymount
to monitor another mount (e.g. /boot)
#!/usr/bin/env python # coding: Latin-1 # Load library functions we want import sys import os import time import re # Parameters to the script defaultMount = '/' # Mount path to observe when none is provided errorColour = '200' # Colour to flash when the mount is not found interval = 1.0 # Delay in seconds between refreshes # Colours from most free to most full driveColour = ['002', '012', '022', '021', '020', '120', '220', '210', '200'] # Function to write the LedBorg colour def SetLedColour(colour): LedBorg = open('/dev/ledborg', 'w') # Open the LedBorg device for writing to LedBorg.write(colour) # Write the colour string to the LedBorg device LedBorg.close() # Close the LedBorg device # Get user input if len(sys.argv) > 1: mountPath = sys.argv[1] else: mountPath = defaultMount print 'Monitoring "' + mountPath + '"' # Set our starting state errorOn = True # Loop indefinitely while True: # Query the disk free program (df) for drive statistics rawDataFile = os.popen('df 2> /dev/null') # The '2> /dev/null' is used to suppress any errors rawData = rawDataFile.readlines() rawDataFile.close() # Parse data looking for our mount used = -1.0 for line in rawData: # Split the raw text into usable chunks line = line[:-1] # Remove last character (newline symbol) line = re.sub(' *', ' ', line) # Replace all instances of multiple spaces with a single space token = line.split(' ') # Split into many strings at the space characters # See if we have the right line if token[5] == mountPath: used = float(token[4][:-1]) # Take the Use%, strip the '%' symbol and convert to a number break # End the search early # Pick our colour and set it if used < 0.0: errorOn = not errorOn # Toggle if the error flash is on or off # Set error flash if errorOn: SetLedColour(errorColour) # Set to error colour else: SetLedColour('000') # Set to off else: used /= 100.0 # Make used between 0 and 1 used *= len(driveColour) # Increase to cover the colour list used = int(used) # Trim to the next integer down if used >= len(driveColour): used = len(driveColour) - 1 # If we had 100% or larger cap at the highest colour SetLedColour(driveColour[used]) # Set the appropriate colour # Wait for the interval time.sleep(interval)
