BashLed - Driving LedBorg directly using Bash
Here is an example for everyone who wants to use an LedBorg without the driver code, or simply wants to see an example of GPIO used in Bash
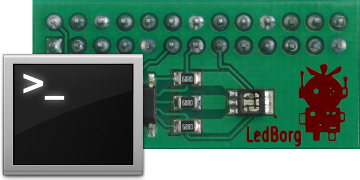
BashLed.sh is a Bash script which uses /sys/class/gpio to set the state of the 3 GPIO pins representing the red, green and blue channels
As an additional bonus it also demonstrates a self elevating script, a script which re-runs itself using sudo if not run as root!
If you have a Rev 1 Raspberry Pi you will need to uncomment (remove the # symbol) from line 5 and comment out (add a # symbol to the beginning) of line 6, otherwise the green channel will not work.
Here's the code, you can download the BashLed script file as text here
Save the text file on your pi as BashLed.sh
If you have the LedBorg driver installed you will need to temporarily disable it to use the GPIO pins instead using
Make the script executable using
and run using
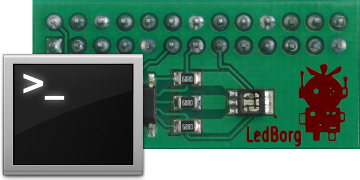
BashLed.sh is a Bash script which uses /sys/class/gpio to set the state of the 3 GPIO pins representing the red, green and blue channels
As an additional bonus it also demonstrates a self elevating script, a script which re-runs itself using sudo if not run as root!
If you have a Rev 1 Raspberry Pi you will need to uncomment (remove the # symbol) from line 5 and comment out (add a # symbol to the beginning) of line 6, otherwise the green channel will not work.
Here's the code, you can download the BashLed script file as text here
Save the text file on your pi as BashLed.sh
If you have the LedBorg driver installed you will need to temporarily disable it to use the GPIO pins instead using
sudo /etc/init.d/ledborg.sh stop
Make the script executable using
chmod +x BashLed.sh
and run using
sudo ./BashLed.sh RGB
#!/bin/bash # GPIO assignments LED_RED=17 #LED_GREEN=21 # Use this line for Rev 1 boards LED_GREEN=27 # Use this line for Rev 2 boards LED_BLUE=22 # Check user ID (run as sudo if not root) if test "$UID" -ne 0 ; then # We are not root, re-run as root echo "Not root, re-running as root" sudo $0 $@ exit 0 fi # Set up GPIO pins as outputs echo "$LED_RED" > /sys/class/gpio/export echo "out" > /sys/class/gpio/gpio${LED_RED}/direction echo "$LED_GREEN" > /sys/class/gpio/export echo "out" > /sys/class/gpio/gpio${LED_GREEN}/direction echo "$LED_BLUE" > /sys/class/gpio/export echo "out" > /sys/class/gpio/gpio${LED_BLUE}/direction # Determine the user colour choice red=`echo $1 | cut -c 1` green=`echo $1 | cut -c 2` blue=`echo $1 | cut -c 3` # Change 2s into 1s if test "$red" -eq 2 ; then red=1 fi if test "$green" -eq 2 ; then green=1 fi if test "$blue" -eq 2 ; then blue=1 fi # Set the colour choice to the GPIO pins if test "$red" -eq 1 ; then echo "1" > /sys/class/gpio/gpio${LED_RED}/value else echo "0" > /sys/class/gpio/gpio${LED_RED}/value fi if test "$green" -eq 1 ; then echo "1" > /sys/class/gpio/gpio${LED_GREEN}/value else echo "0" > /sys/class/gpio/gpio${LED_GREEN}/value fi if test "$blue" -eq 1 ; then echo "1" > /sys/class/gpio/gpio${LED_BLUE}/value else echo "0" > /sys/class/gpio/gpio${LED_BLUE}/value fi # Clean up GPIO pins echo "$LED_RED" > /sys/class/gpio/unexport echo "$LED_GREEN" > /sys/class/gpio/unexport echo "$LED_BLUE" > /sys/class/gpio/unexport
